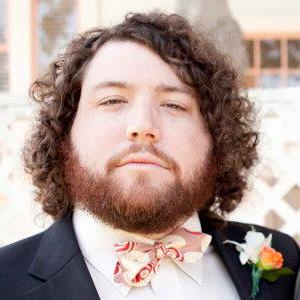
Bootstrap + Lavaca
This tutorial walks through integrating Bootstrap with Lavaca's Starter Application. A sample git repo of this tutorial's end result is available on my GitHub.
Install Bootstrap
Installing dependencies in Lavaca is easy, thanks to Bower. Bower is great for managing client-side dependencies, in-fact Lavaca Starter uses Bower to install Lavaca's core library. To install Boostrap add a new dependency in the bower.json
file.
"dependencies": {
"bootstrap": "~3.0.3",
"lavaca": "latest"
}
Then open up a terminal and cd into the project's root and run:
'$ bower install'
During the install, it may ask what version of jQuery to use. Select the first option to use Lavaca's jQuery version.
Shim Bootstrap for RequireJS
Bootstrap provides some jQuery plugins to breath life into typical UI components like modals, popovers, and tooltips. These components can be shimmed individually or all at once. For simplicity lets shim the entire library.
In order for Bootstrap's JavaScript components to play nice with jQuery in a RequireJS setting we need to shim in the library. Open src/www/js/app/boot.js
and add a path and shim entry.
paths: {
...
'bootstrap': '../components/bootstrap/dist/js/bootstrap'
},
shim: {
...
bootstrap: {
deps: ['$']
}
}
This adds an alias for bootstrap's path to the components directory. The bootstrap module is now available by simply requiring it like require('bootstrap')
. The shim entry allows us to define jQuery as a dependency of bootstrap, which prevents bootstrap from loading before jQuery.
The final step in adding Bootstrap's JavaScript components to our project is requiring the module. Since bootstrap just adds plugins to the jQuery namespace, we only need to require the module once. A logical place to add the module is in src/www/js/app/app.js
.
define(function(require) {
var History = require('lavaca/net/History');
var HomeController = require('./net/HomeController');
var Connectivity = require('lavaca/net/Connectivity');
var Application = require('lavaca/mvc/Application');
var Translation = require('lavaca/util/Translation');
var headerView = require('app/ui/views/controls/HeaderView');
require('lavaca/ui/DustTemplate');
require('hammer');
require('bootstrap'); // <-- Adds bootstrap's js components
...
To LESS or not to LESS
Lavaca Starter uses LESS out of the Box. Bootstrap gives the option of using LESS or compiled CSS. The LESS option is recommended since it is the most flexible and fits nicely into a typical Lavaca app workflow.
To use Bootstrap's LESS files, just import them into src/www/css/app/app.less
.
@import '../libs/libs.less';
@import '../../components/bootstrap/less/bootstrap.less';
@import '../../components/bootstrap/less/theme.less';
...
That's it!
If you don't plan to theme or make use of the LESS variables you can simply link components/bootstrap/dist/css/bootstrap-min.css
and components/bootstrap/dist/css/bootstrap-theme-min.css
into src/www/index.html
. The only gotcha here is the components directory isn't copied by default during the build process, so your app won't work unless you alter the Gruntfile.js
.
copy: {
www: [
'<%= paths.out.index %>',
'<%= paths.out.css %>/<%= package.name %>.css ',
'<%= paths.out.js %>/<%= package.name %>.min.js',
'configs/**/*',
'assets/**/*',
'messages/**/*',
'config.xml',
'components/bootstrap/dist/**/*' // <-- Add boostrap dist directory to copy task
]
}
Conclusion
The default styles for HeaderView
and HomeView
are not really bootstrap friendly, so you may see the Lavaca logo is misaligned when you run grunt server
. These styles are meant to throwaway anyways, so feel free to dump them and start over.
Questions or Feedback? Hit me up in the comments or on twitter @wghenderson